My second BASIC to C conversion using the classic Usborne 1980s computer books which helped many of today’s tech professionals were inspired by the Usborne computing books they read as children. The books included program listings for such iconic computers as the ZX Spectrum, the BBC Micro and the Commodore 64, and are still used in some computer clubs today.
Usborne books still continue to publish books on many topics including programming using modern languages but on their website they also allow the old classic books to be downloaded for free, so if you want to play around with them click here.
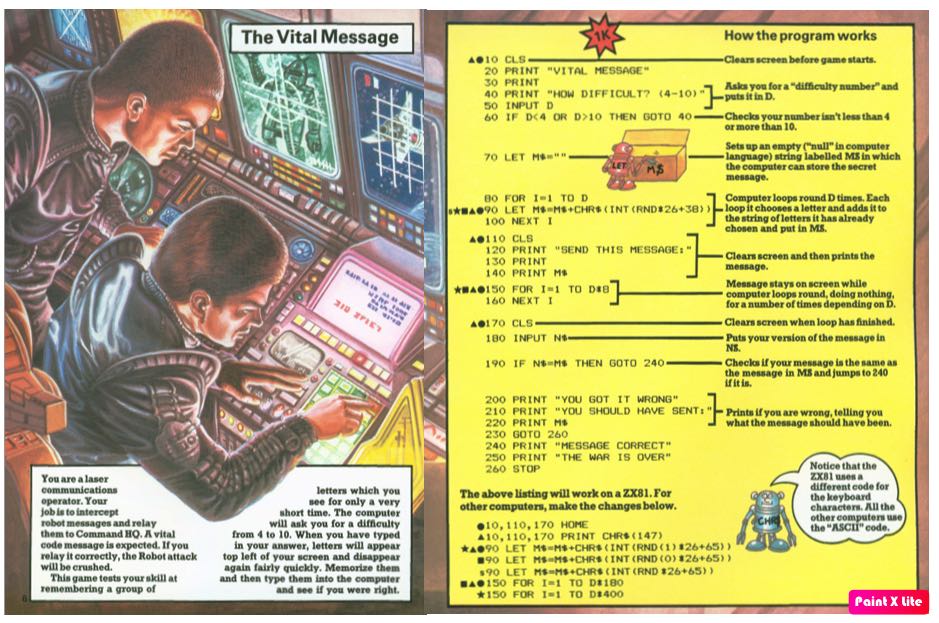
/*
The Vital Message
You are a laser communications operator. Your job is to intercept robot messages and relay them to Command HQ. A vitial code message is expected. If you relay it correctly, the Robot attack will be crushed.
This game tests your skill at remembering a group of letters which you see for only a very short time. The computer will ask you for a difficulty from 4 to 10. When you have typed in your answer, letters will appear top left of your screen and disappear again fairly quickly. memorise them and then type them into the computer and see if you were right.
Compiler information: gcc -o vitial vital.c
*/
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <ctype.h>
#include <string.h>
void exit(int status);
int main() {
char robotCode[10] = "";
char playerEntry[10] = "";
int difficulty = 0;
int iCounter = 0;
time_t t;
time_t start, end;
system("clear");
printf("Vital Message\n\n");
printf("How difficult? (4 - 10) ?");
scanf(" %d", &difficulty);
if (( difficulty <= 4 ) && difficulty >= 10) {
difficulty = 4; // Set default 4 character message
}
while (iCounter < difficulty) {
srand((unsigned) time(&t)+iCounter);
robotCode[iCounter] = 65 + rand() % 26;
iCounter++;
}
system("clear");
printf("Send this message: ");
printf(" %s\n\n", robotCode);
time(&start);
do time(&end); while(difftime(end, start) <= difficulty/2);
system("clear");
printf("Enter the code: ");
scanf(" %s", playerEntry);
if (strcmp(robotCode, playerEntry) == 0) {
printf("Message correct\n");
printf("The war is over\n\n");
exit(0);
} else {
printf("You got it wrong\n");
printf("You should have sent:\n\n");
printf(" %s\n\n",robotCode);
}
return 0;
}